Developing Android apps is a wonderful way to boost your company’s performance, but deciding which programming language to use is the actual challenge. For many of us, it is self-evident that Java is the finest since it is widely used and praised. However, with the introduction of Kotlin, programmers’ seeming faith in Java is shattered, and they begin looking for answers to questions like:
- Is Kotlin better than Java?
- Kotlin vs Java for Android Development?
We decided to produce this article in order to help you understand the differences between Kotlin and Java. Here, we’ll compare and contrast Kotlin vs Java for Android development.
So let’s get this ball rolling!
Java removes boilerplate code and makes code highly expressive, and intuitive—easy to both write and maintain.
What is Java?
Android’s native language is Java, which is used by apps that interface with the operating system and directly utilize the hardware. This language permits the construction of any application and is compatible with nearly all computers and operating systems, including Android, Windows, and Linux. Microservices may be used with Java, which was created by Sun Microsystems (now owned by Oracle).
What Are Java’s Advantages?
The advantages of the Java programming language are listed below.
- Java is cross-platform, meaning it can run on almost any device, server, or operating system.
- Its coding is strong, and Java instructions cannot damage memory or jeopardize data from other OS X programmes.
- Because Java is object-oriented, it is simple to develop modular programmes and reuse components that contribute to the robustness of the system.
- It’s ready to use, and with Java, you’ll receive a lot of ready-to-use third-party code.
- When comparing the performance of Kotlin and Java to that of other languages, Java is easier to use, compile, debug, and deploy.
- Because many of its libraries are handled by reputable organizations like Google, Apache, and others, Java is an open-source language that assures security.
What are the Challenges of the Java language?
The following are some of Java’s difficulties.
- When compared to other languages like C + or Python, Java’s syntax might be a touch complex or tedious.
- It’s impossible to access material that’s incompatible with the device or equipment you’re using Java.
- It’s not simple to get your hands on the latest Java improvements for mobile development.
- There are occasions when Java interferes with the design of Android APIs.
- Test-Driven Development for Java necessitates the creation of additional code and increases the likelihood of programming mistakes and defects.
- Java is slightly slower than other programming languages and consumes a significant amount of system memory.
What are the Applications of Java?
- Spotify
- Cash App
- Signal
- Amaze File Manager
What is Kotlin?
When it comes to Kotlin vs. Java, Kotlin is a recently developed language that is based on Java, however, it is a more advanced version with many more capabilities. In comparison to Java and other programming languages, it is clean, straightforward, and has fewer formalities and conventions. To utilize this language to create Android apps, developers must first master the fundamentals of programming.
What are the Benefits of Kotlin?
- Kotlin Application Deployment is a lightweight, fast-compiling language that keeps applications from growing in size.
- When compared to Java, each block of code produced in Kotlin is significantly smaller, as it is less verbose, and less code equals fewer errors.
- Kotlin compiles the code into bytecode that can be run on the Java Virtual Machine (JVM). As a result, all Java libraries and frameworks transfer and execute in a Kotlin project.
- Kotlin has a steep learning curve, and moving teams to Kotlin might be difficult due to the language’s compact syntax.
- In the market, there are just a few Kotlin developers. As a result, finding an experienced mentor might be difficult.
What are the Applications of Kotlin?
- Trello
- Evernote
- Twidere for Twitter
- Shadowsocks
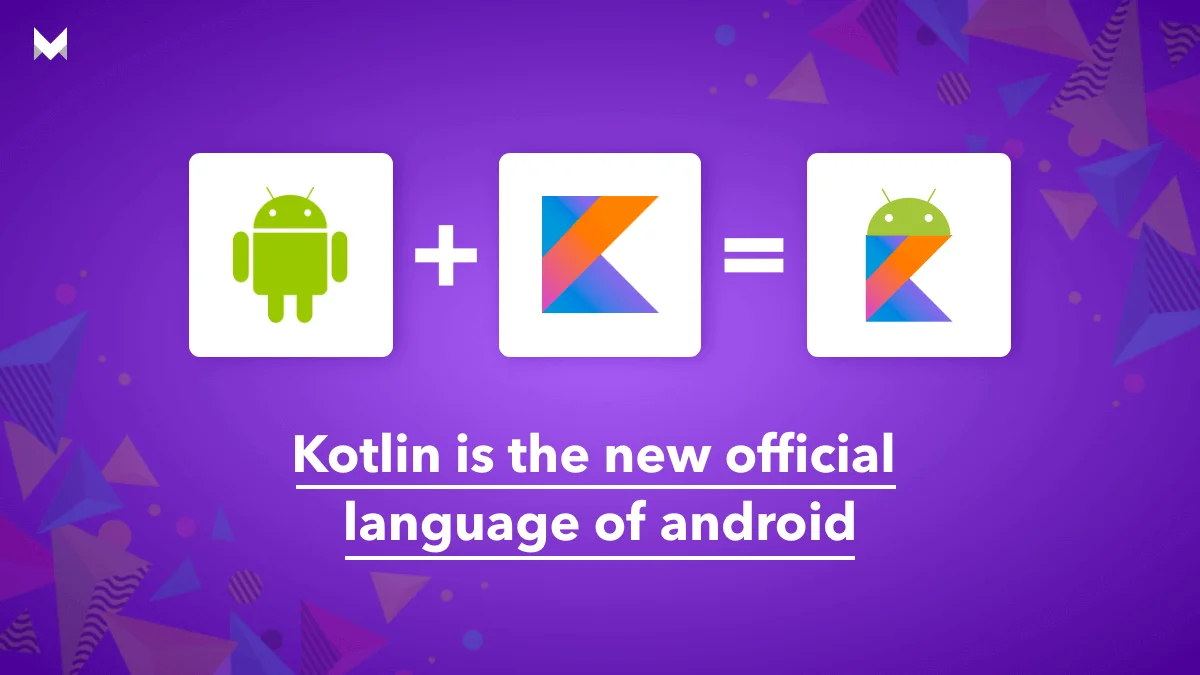
A Quick Difference between Kotlin and Java
Despite the fact that Kotlin is an officially approved language for writing Android apps, you may still be hesitant to make the transition. Why should you change when Java has been shown to work for all of these?
Here are some of the reasons why relocating to Kotlin is one of the finest decisions you can make.
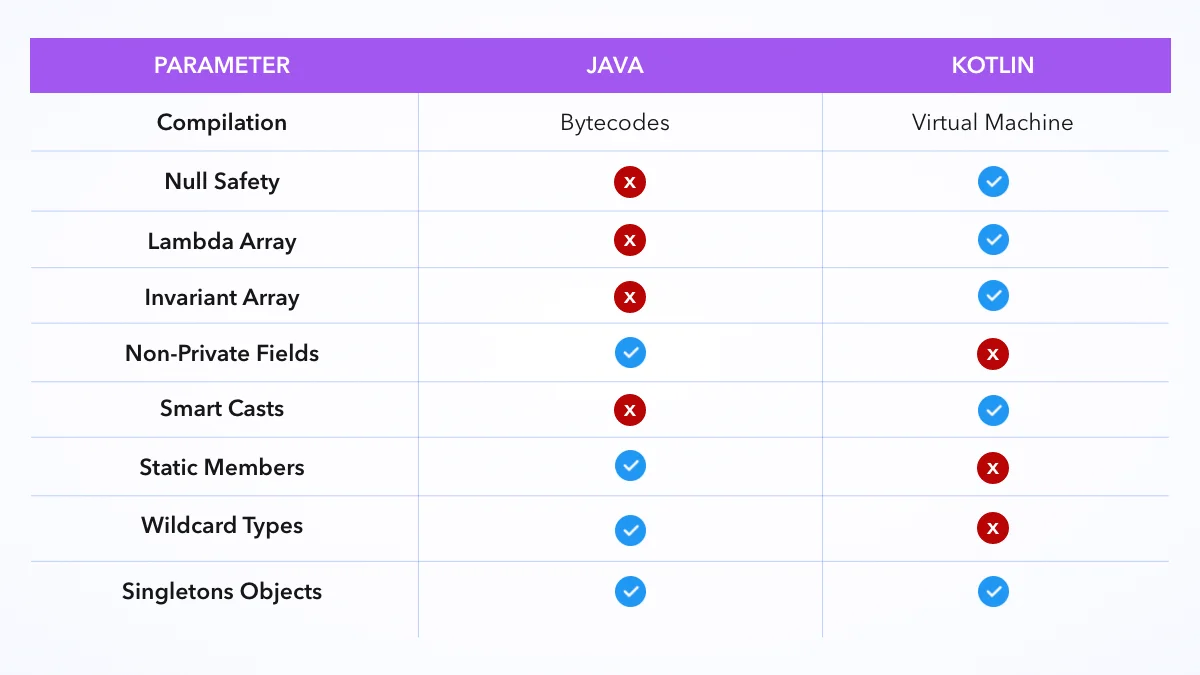
Kotlin vs Java – Feature Showdown With Syntax
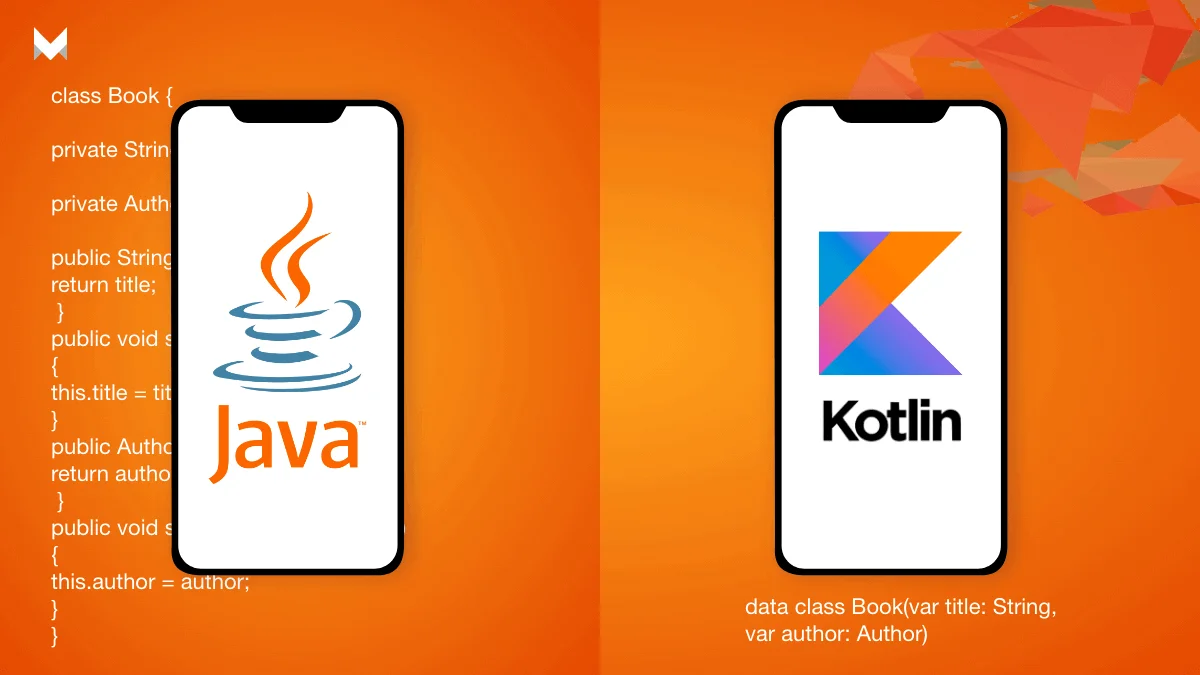
Null Safety
NullPointerException, often known as NPE, is one of Java’s major flaws, and the only conceivable cause is an intentional request to throw NullPointerException. Some of the data inconsistencies were caused by difficulties with initialization or by external Java code.
As discussed in the previous section, Kotlin avoids NullPointerException when compared to Java. When a NullPointerException is raised, Kotlin fails at compile time.
Data Classes
When comparing Java vs Kotlin for Android, Data Classes are used to generate boilerplate such as equals, hashCode, function toString, getters/setters, and more. Consider the following illustration:
/* Java Code */
class Book {
private String title;
private Author author;
public String getTitle() {
return title;
}
public void setTitle(String title)
{
this.title = title;
}
public Author getAuthor() {
return author;
}
public void setAuthor(Author author)
{
this.author = author;
}
}
However, in Kotlin, the same above class may be defined in a single line —
/* kotlin Code */
data class Book(var title: String,
var author: Author)
Functions of Extensions
We can enhance the functionality of existing classes in Kotlin without having to inherit from them. To put it another way, in comparison to Java, Kotlin allows you to build a class with new functionality without needing to inherit from it. This is accomplished using extension functions.
fun MutableList < Int> .swap(index1: Int, index2: Int) {
val tmp = this[index1]
this[index1] = this[index2]
this[index2] = tmp
}
The receiver object, given before the dot, is referred to by the ‘this’ keyword inside the extension function. We can now call a method like this on any MutableList —
val abc = mutableListOf(1, 2, 3)
abc.swap(0, 2)
Smart Casts
Kotlin’s compiler is clever when it comes to casting. In many situations, explicit cast operators are not required in Kotlin; nonetheless, when comparing Kotlin to Java, Kotlin does “is-checks” for immutable data and inserts cast automatically where necessary .
fun demo(x: Any) {
if (x is String) {
print(x.length) // x is automatically cast to string
}
}
Inference of Type
The fact that you don’t have to define the type of each variable directly in Kotlin is a huge plus (in clear and detailed manner). However, if you wish to explicitly specify a data type in Kotlin versus Java Android, you may do so. Consider the following situation:
/* not explicitly defined */
fun main(args: Array < String> ) {
val text = 10
println(text)
}
/* explicitly defined */
fun main(args: Array < String> ) {
val text: Int = 10
println(text)
}
Functional Programming
The fact that Kotlin is a functional programming language is the most crucial factor in its performance when compared to Java. Higher-order functions, lambda expressions, operator overloading, slow evaluation, operator overloading, and much more are among the many useful methods in Kotlin.
When it comes to collections, Functional Programming makes Kotlin a lot more useful —
fun main(args: Array < String> ) {
val numbers = arrayListOf(15, -5, 11, -39)
val nonNegativeNumbers = numbers.filter
{
it >= 0
}
println(nonNegativeNumbers)
}
Output – 15, 11
Higher-Order Functions are functions that accept a function as a parameter and also returns a function. Take a look at the following code:
fun alphaNum(func: () -> Unit) {}
In the above code “func” is the name of the parameter and “ ( ) -> Unit ” is the function type. In this case, we are saying that func will be a function that does not receive any parameter and does not return any value also.
Lambda expression or an anonymous function is a “function literal”, i.e. a function that is not declared but passed immediately as an expression.
An Example of a Lambda Expression –
val sum: (Int, Int) – > Int = {
x,
y – > x + y
}
We just declare a variable ‘sum’ that takes two numbers and adds them together, returning total as an integer in the example above.
To call it, we just use the expression ‘sum(2,2)’.
Isn’t it cool?
In Kotlin versus Java Android, an anonymous function is one that allows us to define the return type while omitting the function name. Take the following scenario as an example:
Either this way:
fun(x: Int, y: Int): Int = x + y
or This Way
fun(x: Int, y: int): Int {
return a + b
}
So, who ascended to the throne?
Obviously, Kotlin.
This is because Kotlin integrates with Java, allowing for incremental code changes and a superior type system over Java, as well as a simple migration path from Java with backward compatibility.
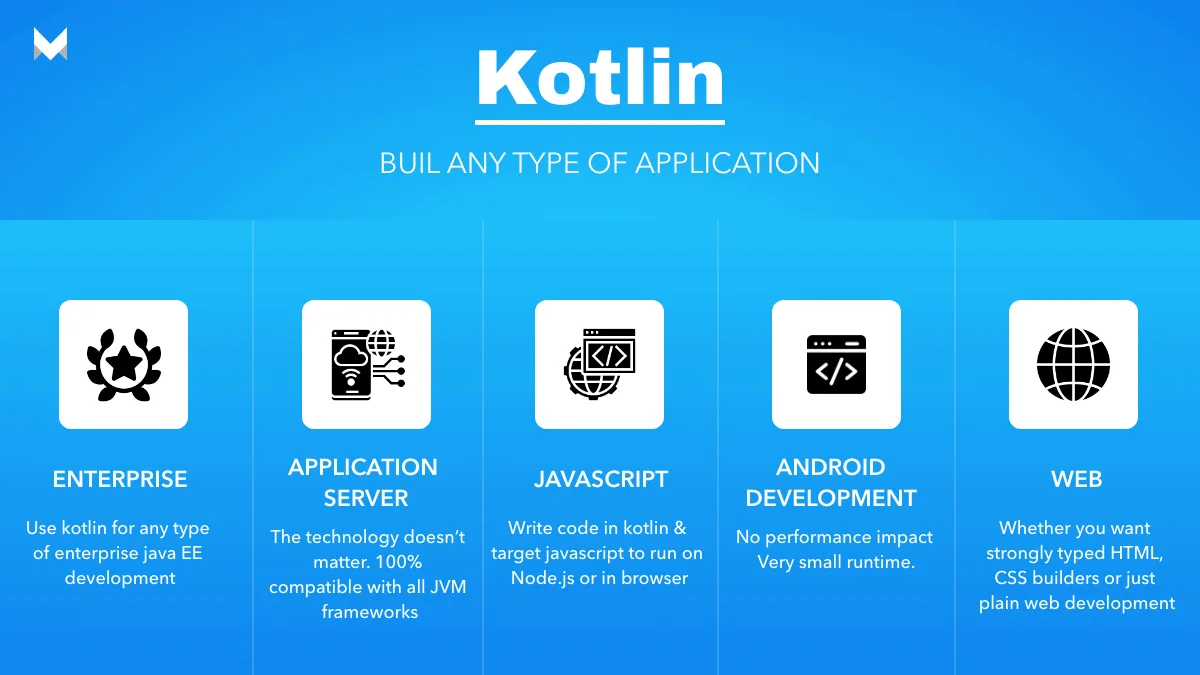
Putting It All Together
Mobcoder, a mobile app development company, believes that the time it takes for incremental builds to compile is more important to us than the time it takes for clean builds. So, in terms of Kotlin vs. Java for android app development, Kotlin is identical to Java, and yes, we can use Kotlin without having to worry about compiling time.